Often I need to load the content of a file into a Windows Command Prompt variable. This is useful when writing batch scripts where the return value from one program is used as input for another program. At other times I need to process a json file to load just a value into a variable.
Note: There is a limit to the size of variables. I think the limit is 1024 bytes.
Let’s start with a sample test file “testfile”:
1 2 3 |
This is a test file line 1 This is a test file line 2 This is a test file line 3 |
And another sample test file “testfile.json”:
1 2 3 4 5 6 7 |
{ "creationTimestamp": "2018-10-25T16:31:48.172-07:00", "description": "", "id": "6381188456147577995", "kind": "compute#instanceTemplate", "name": "instance-template-1" } |
Load the first line from testfile into a variable:
1 2 3 |
REM - load the first line from testfile set /p T=<testfile echo %T% |
This outputs:
1 |
This is a test file line 1 |
Load the last line from testfile into a variable:
1 2 3 |
REM - load the last line from testfile for /f "delims=" %%x in (testfile) do set T=%%x echo %T% |
This outputs:
1 |
This is a test file line 3 |
Load all lines from testfile.json into a variable:
1 2 3 4 5 |
REM - load all of the lines from testfile.json set T= SETLOCAL EnableDelayedExpansion for /f "Tokens=* Delims=" %%x in (testfile.json) do set T=!T!%%x echo %T% |
This outputs:
1 |
{ "creationTimestamp": "2018-10-25T16:31:48.172-07:00", "description": "", "id": "6381188456147577995", "kind": "compute#instanceTemplate", "name": "instance-template-1"} |
Load just a value from a json file:
1 2 3 4 |
REM - load just a value from a json file jq .name testfile.json > out.tmp set /p T=<out.tmp echo %T% |
This outputs:
1 |
"instance-template-1" |
Load just a value from a json file without the quotes:
1 2 3 4 |
REM - load just a value from a json file without quotes jq -r .name testfile.json > out.tmp set /p T=<out.tmp echo %T% |
This outputs:
1 |
instance-template-1 |
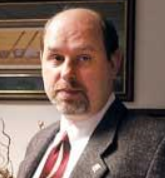
I design software for enterprise-class systems and data centers. My background is 30+ years in storage (SCSI, FC, iSCSI, disk arrays, imaging) virtualization. 20+ years in identity, security, and forensics.
For the past 14+ years, I have been working in the cloud (AWS, Azure, Google, Alibaba, IBM, Oracle) designing hybrid and multi-cloud software solutions. I am an MVP/GDE with several.
Leave a Reply