I have worked with Google Cloud Stackdriver for about three months. The more I learn about Stackdriver the more I like it. Great product for logging, monitoring, error reporting, application tracing and application debugging and more.
One of the items that I don’t like with web server uptime checks is that the health checks clutter up my server’s log files. I want to know what IP addresses Stackdriver agents use so that I can filter my log files.
This week I learned about a new Google Cloud beta feature that supports listing Stackdriver IP addresses: Getting uptime-check IP addresses
The following code in Python will generate a table with information about the IP addresses that Stackdriver agents use.
1 2 3 4 5 6 7 8 9 10 11 |
from google.cloud import monitoring_v3 import tabulate client = monitoring_v3.UptimeCheckServiceClient() ips = client.list_uptime_check_ips() print(tabulate.tabulate( [(ip.region, ip.location, ip.ip_address) for ip in ips], ('region', 'location', 'ip_address') )) |
This results in a table similar to the following.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
region location ip_address -------- ----------------- --------------- 1 Oregon 35.197.117.125 1 Oregon 35.203.157.42 1 Oregon 35.199.157.7 1 Oregon 35.233.206.171 1 Oregon 35.197.32.224 1 Oregon 35.233.167.246 1 Oregon 35.203.129.73 1 Oregon 35.185.252.44 1 Oregon 35.233.165.146 1 Virginia 35.186.164.184 1 Virginia 35.188.230.101 1 Virginia 35.199.27.30 1 Virginia 35.186.176.31 1 Virginia 35.236.207.68 1 Virginia 35.236.221.2 1 Virginia 35.221.55.249 1 Virginia 35.199.12.162 1 Virginia 35.186.167.85 1 Iowa 146.148.59.114 1 Iowa 23.251.144.62 1 Iowa 146.148.41.163 1 Iowa 35.239.194.85 1 Iowa 104.197.30.241 1 Iowa 35.192.92.84 1 Iowa 35.238.3.7 1 Iowa 35.224.249.156 1 Iowa 35.238.118.210 3 Sao Paulo, Brazil 35.199.66.47 3 Sao Paulo, Brazil 35.198.18.224 3 Sao Paulo, Brazil 35.199.67.79 3 Sao Paulo, Brazil 35.198.36.209 3 Sao Paulo, Brazil 35.199.90.14 3 Sao Paulo, Brazil 35.199.123.150 3 Sao Paulo, Brazil 35.198.39.162 3 Sao Paulo, Brazil 35.199.77.186 3 Sao Paulo, Brazil 35.199.126.168 2 Belgium 104.155.77.122 2 Belgium 104.155.110.139 2 Belgium 146.148.119.250 2 Belgium 35.195.128.75 2 Belgium 35.240.124.58 2 Belgium 35.205.234.10 2 Belgium 35.205.72.231 2 Belgium 35.187.114.193 2 Belgium 35.205.205.242 4 Singapore 35.187.242.246 4 Singapore 35.186.144.97 4 Singapore 35.198.221.49 4 Singapore 35.198.194.122 4 Singapore 35.198.248.66 4 Singapore 35.185.178.105 4 Singapore 35.198.224.104 4 Singapore 35.240.151.105 4 Singapore 35.186.159.51 |
By modifying the Python code, you can generate the list of IP addresses in any format required to filter your log files.
The following code in c# does the same thing. Notice that my code requires the beta03 dotnet libraries. This is a new feature from Google Cloud.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
using System; using Google.Cloud.Monitoring.V3; // Installation as of Dec 18, 2018 - requires beta version // dotnet add package Google.Cloud.Monitoring.V3 --version 1.1.0-beta03 namespace addr { class Program { static void Main(string[] args) { var client = UptimeCheckServiceClient.Create(); var ips = client.ListUptimeCheckIps(new ListUptimeCheckIpsRequest()); Console.WriteLine("{0,-20} {1,-20} {2}", "Region", "Location", "IpAddress"); Console.WriteLine("-------------------- -------------------- -------------------"); foreach (UptimeCheckIp ip in ips) { Console.WriteLine("{0,-20} {1,-20} {2}", ip.Region, ip.Location, ip.IpAddress); } } } } |
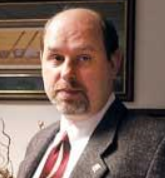
I design software for enterprise-class systems and data centers. My background is 30+ years in storage (SCSI, FC, iSCSI, disk arrays, imaging) virtualization. 20+ years in identity, security, and forensics.
For the past 14+ years, I have been working in the cloud (AWS, Azure, Google, Alibaba, IBM, Oracle) designing hybrid and multi-cloud software solutions. I am an MVP/GDE with several.
Leave a Reply