This article shows how to display a list of Google Cloud Projects that you have access to list. This article includes two examples in Python that use two different Google Cloud Python libraries. These examples produce the same output as the Google CLI gcloud projects list
command.
You can only list projects for which you have permissions to access. This means that you cannot see all projects unless you have the rights to access them. In my examples below I show which scopes are required. This also means that you can list projects across accounts. This allows you to see which projects you have access to using the credentials specified in the examples. I show how to use Application Default Credentials (ADC) and Service Account Credentials (Json file format).
These examples have been tested with Python 3.6 on Windows 10 Professional.
Example 1 using the Python Client Library (services discovery method):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
from googleapiclient import discovery from oauth2client.client import GoogleCredentials from google.oauth2 import service_account # Example using the Python Client Library # Documentation # https://github.com/googleapis/google-api-python-client # https://developers.google.com/resources/api-libraries/documentation/cloudresourcemanager/v2/python/latest/ # Library Installation # pip install -U google-api-python-client # pip install -U oauth2client # Requires one of the following scopes # https://www.googleapis.com/auth/cloud-platform # https://www.googleapis.com/auth/cloud-platform.read-only # https://www.googleapis.com/auth/cloudplatformprojects # https://www.googleapis.com/auth/cloudplatformprojects.readonly print('{:<20} {:<22} {:<21}'.format('PROJECT_ID', 'NAME', 'PROJECT_NUMBER')) # Uncomment to use Application Default Credentials (ADC) credentials = GoogleCredentials.get_application_default() # Uncomment to use Service Account Credentials in Json format # credentials = service_account.Credentials.from_service_account_file('service-account.json') service = discovery.build('cloudresourcemanager', 'v1', credentials=credentials) request = service.projects().list() while request is not None: response = request.execute() for project in response.get('projects', []): print('{:<20} {:<22} {:<21}'.format(project['projectId'], project['name'], project['projectNumber'])) request = service.projects().list_next(previous_request=request, previous_response=response) |
Example 2 using the Python Google Cloud Resource Manager API Client Library:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
from google.cloud import resource_manager # Example using the Python Google Cloud Resource Manager API Client Library # Documentation # https://pypi.org/project/google-cloud-resource-manager/ # https://github.com/googleapis/google-cloud-python # https://googleapis.github.io/google-cloud-python/latest/resource-manager/index.html # https://googleapis.github.io/google-cloud-python/latest/resource-manager/client.html # https://googleapis.github.io/google-cloud-python/latest/resource-manager/project.html # Library Installation # pip install -U google-cloud-resource-manager # Requires one of the following scopes # https://www.googleapis.com/auth/cloud-platform # https://www.googleapis.com/auth/cloud-platform.read-only # https://www.googleapis.com/auth/cloudplatformprojects # https://www.googleapis.com/auth/cloudplatformprojects.readonly print('{:<20} {:<22} {:<21}'.format('PROJECT_ID', 'NAME', 'PROJECT_NUMBER')) # Uncomment to use Application Default Credentials (ADC) client = resource_manager.Client() # Uncomment to use Service Account Credentials in Json format # client = resource_manager.Client.from_service_account_json('service-account.json') for project in client.list_projects(): print('{:<20} {:<22} {:<21}'.format(project.project_id, project.name, project.number)) |
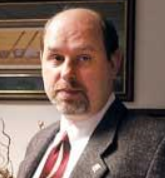
I design software for enterprise-class systems and data centers. My background is 30+ years in storage (SCSI, FC, iSCSI, disk arrays, imaging) virtualization. 20+ years in identity, security, and forensics.
For the past 14+ years, I have been working in the cloud (AWS, Azure, Google, Alibaba, IBM, Oracle) designing hybrid and multi-cloud software solutions. I am an MVP/GDE with several.
Leave a Reply